要在Vue项目中为视频添加文字,可以通过以下几个步骤实现:1、使用HTML5的
一、加载视频
首先,你需要在Vue组件中加载视频文件。使用HTML5的
<template>
<div>
<video ref="videoPlayer" width="600" controls>
<source src="your-video-file.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
</div>
</template>
在这个示例中,我们使用了
二、定位文字
为了在视频上添加文字,我们需要使用CSS样式来定位文字。可以通过绝对定位将文字放置在视频的特定位置。下面是一个示例代码:
<template>
<div>
<video ref="videoPlayer" width="600" controls>
<source src="your-video-file.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
<div class="video-overlay-text" v-if="showText">
{{ overlayText }}
</div>
</div>
</template>
<style>
.video-overlay-text {
position: absolute;
top: 50px; /* 根据需要调整位置 */
left: 50px; /* 根据需要调整位置 */
color: white;
background-color: rgba(0, 0, 0, 0.5); /* 半透明背景 */
padding: 5px;
border-radius: 5px;
}
</style>
在这个示例中,我们创建了一个
三、控制文字显示
接下来,我们使用Vue的数据绑定和方法来控制文字的显示。可以通过一个布尔值来控制文字的显示与隐藏,并通过一个字符串来动态更新文字内容。下面是一个示例代码:
<script>
export default {
data() {
return {
showText: false,
overlayText: 'Initial Text'
};
},
methods: {
displayText(newText) {
this.overlayText = newText;
this.showText = true;
setTimeout(() => {
this.showText = false;
}, 3000); // 文字显示3秒后隐藏
}
}
};
</script>
在这个示例中,我们定义了两个数据属性:showText和overlayText。showText用于控制文字的显示与隐藏,overlayText用于存储要显示的文字内容。我们还定义了一个方法displayText,用于更新文字内容并显示文字。
四、触发文字显示
最后,我们需要在适当的时间触发文字显示。例如,当视频播放到特定时间时显示文字。可以通过监听
<template>
<div>
<video ref="videoPlayer" width="600" controls @timeupdate="onTimeUpdate">
<source src="your-video-file.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
<div class="video-overlay-text" v-if="showText">
{{ overlayText }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
showText: false,
overlayText: 'Initial Text'
};
},
methods: {
displayText(newText) {
this.overlayText = newText;
this.showText = true;
setTimeout(() => {
this.showText = false;
}, 3000); // 文字显示3秒后隐藏
},
onTimeUpdate() {
const currentTime = this.$refs.videoPlayer.currentTime;
// 根据视频播放时间触发文字显示
if (currentTime > 5 && currentTime < 6) {
this.displayText('Text at 5 seconds');
}
}
}
};
</script>
在这个示例中,我们监听了
通过以上步骤,你就可以在Vue项目中为视频添加文字了。总结一下,主要步骤包括:1、加载视频;2、定位文字;3、控制文字显示;4、触发文字显示。希望这些内容对你有帮助。如果你有进一步的问题或需求,可以继续深入学习和调整代码以满足特定需求。
相关问答FAQs:
1. 如何在Vue中实现在视频中加字的效果?
在Vue中,可以使用video.js这个流行的HTML5视频播放器库来实现在视频中加字的效果。首先,你需要在Vue项目中安装video.js和相应的插件。
步骤如下:
- 安装video.js和videojs-contrib-hls插件。在命令行中运行以下命令:
npm install video.js videojs-contrib-hls
- 在Vue组件中引入video.js和相应的样式文件。在你的组件中添加以下代码:
import videojs from 'video.js';
import 'video.js/dist/video-js.css';
- 在组件的mounted钩子函数中,初始化video.js播放器并添加字幕。以下是一个示例代码:
mounted() {
// 创建video元素
const videoElement = document.createElement('video');
this.$el.appendChild(videoElement);
// 初始化video.js播放器
const player = videojs(videoElement);
// 添加字幕
player.addRemoteTextTrack({
kind: 'captions',
src: 'path/to/captions.vtt',
srclang: 'en',
label: 'English',
});
}
请注意,上述代码中的'path/to/captions.vtt'是你的字幕文件的路径。你需要将其替换为你实际的字幕文件路径。
- 在Vue组件销毁前,记得销毁video.js播放器以释放资源。在组件的beforeDestroy钩子函数中添加以下代码:
beforeDestroy() {
// 销毁video.js播放器
if (this.player) {
this.player.dispose();
}
}
这样,你就可以在Vue项目中实现在视频中加字的效果了。
2. Vue中有没有现成的插件可以用来在视频中加字?
是的,Vue中有一些现成的插件可以用来在视频中加字。
其中一个流行的插件是Vue Video Player。它是基于video.js开发的,提供了丰富的功能和易于使用的API。
使用Vue Video Player,你可以轻松地在Vue项目中实现在视频中加字的效果。以下是一个基本的示例:
<template>
<div>
<vue-video-player ref="player" :options="playerOptions"></vue-video-player>
</div>
</template>
<script>
import VueVideoPlayer from 'vue-video-player';
import 'video.js/dist/video-js.css';
export default {
components: {
VueVideoPlayer,
},
data() {
return {
playerOptions: {
sources: [{
src: 'path/to/video.mp4',
type: 'video/mp4',
}],
tracks: [{
kind: 'captions',
src: 'path/to/captions.vtt',
srclang: 'en',
label: 'English',
}],
},
};
},
};
</script>
在上述代码中,'path/to/video.mp4'是你的视频文件路径,'path/to/captions.vtt'是你的字幕文件路径。你需要将它们替换为你实际的文件路径。
通过使用Vue Video Player,你可以轻松地在Vue项目中实现在视频中加字的效果。
3. 如何在Vue中实现自定义样式的视频字幕?
在Vue中,你可以通过使用CSS来自定义样式的视频字幕。以下是一个简单的示例:
<template>
<div>
<video ref="video" controls>
<track src="path/to/captions.vtt" kind="captions" srclang="en" label="English" default>
<source src="path/to/video.mp4" type="video/mp4">
</video>
</div>
</template>
<script>
export default {
mounted() {
const videoElement = this.$refs.video;
const tracks = videoElement.textTracks[0];
tracks.mode = 'showing';
tracks.addEventListener('cuechange', () => {
const activeCue = tracks.activeCues[0];
if (activeCue) {
// 自定义字幕样式
activeCue.getCueAsHTML().style.color = 'red';
activeCue.getCueAsHTML().style.fontSize = '20px';
}
});
},
};
</script>
在上述代码中,'path/to/video.mp4'是你的视频文件路径,'path/to/captions.vtt'是你的字幕文件路径。你需要将它们替换为你实际的文件路径。
通过在Vue组件的mounted钩子函数中添加相应的代码,你可以自定义样式的视频字幕,例如改变字体颜色、字体大小等。
这样,你就可以在Vue项目中实现自定义样式的视频字幕了。
文章标题:vue如何在视频中加字,发布者:飞飞,转载请注明出处:https://worktile.com/kb/p/3642227
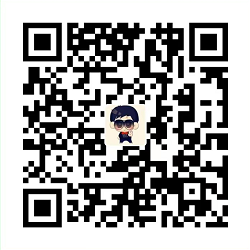
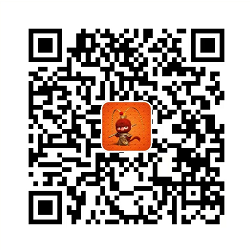