在Vue中,可以通过组合HTML和CSS来实现图片下方添加文字的效果。1、使用<figure>
和<figcaption>
标签,2、利用<div>
和<span>
标签,3、通过CSS定位和样式控制,下面是详细的步骤和示例代码。
一、使用`
`和``标签
使用<figure>
和<figcaption>
标签是一种语义化的方式,可以将图片和文字进行关联,非常适合用于展示图文信息。
<template>
<figure>
<img src="path/to/your/image.jpg" alt="描述图片的文字">
<figcaption>这是图片下方的文字说明</figcaption>
</figure>
</template>
<style scoped>
figure {
text-align: center;
margin: 20px;
}
img {
width: 100%;
height: auto;
}
figcaption {
margin-top: 10px;
font-size: 16px;
color: #555;
}
</style>
二、利用`
`和``标签
如果需要更复杂的布局和样式控制,可以使用<div>
和<span>
标签,通过CSS进行样式调整。
<template>
<div class="image-container">
<img src="path/to/your/image.jpg" alt="描述图片的文字">
<span class="image-caption">这是图片下方的文字说明</span>
</div>
</template>
<style scoped>
.image-container {
text-align: center;
margin: 20px;
position: relative;
}
.image-caption {
display: block;
margin-top: 10px;
font-size: 16px;
color: #555;
}
</style>
三、通过CSS定位和样式控制
在需要更复杂的布局时,可以使用CSS定位和样式控制,例如绝对定位或Flexbox布局。
<template>
<div class="image-wrapper">
<img src="path/to/your/image.jpg" alt="描述图片的文字">
<div class="caption">这是图片下方的文字说明</div>
</div>
</template>
<style scoped>
.image-wrapper {
position: relative;
text-align: center;
margin: 20px;
}
img {
width: 100%;
height: auto;
}
.caption {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
background-color: rgba(0, 0, 0, 0.5);
color: #fff;
padding: 5px 10px;
border-radius: 5px;
}
</style>
四、使用Vue组件化处理
为了更好的复用和维护,可以将图文显示的部分封装成一个Vue组件。
<template>
<div class="image-text">
<img :src="imageSrc" :alt="imageAlt">
<p>{{ caption }}</p>
</div>
</template>
<script>
export default {
props: {
imageSrc: {
type: String,
required: true
},
imageAlt: {
type: String,
default: ''
},
caption: {
type: String,
required: true
}
}
}
</script>
<style scoped>
.image-text {
text-align: center;
margin: 20px;
}
img {
width: 100%;
height: auto;
}
p {
margin-top: 10px;
font-size: 16px;
color: #555;
}
</style>
使用这个组件时,可以在父组件中传递图片和文字的参数:
<template>
<div>
<ImageText
imageSrc="path/to/your/image.jpg"
imageAlt="描述图片的文字"
caption="这是图片下方的文字说明"
/>
</div>
</template>
<script>
import ImageText from './components/ImageText.vue';
export default {
components: {
ImageText
}
}
</script>
总结:在Vue中实现图片下方添加文字,可以通过使用<figure>
和<figcaption>
标签、利用<div>
和<span>
标签、以及通过CSS定位和样式控制等方式来实现。选择合适的方法可以根据具体需求和项目的复杂度来决定。为了更好的代码复用性和可维护性,可以将图文显示部分封装成Vue组件。
相关问答FAQs:
1. 如何在Vue中实现图片下方添加文字效果?
在Vue中,要实现在图片下方添加文字的效果,可以通过以下步骤进行操作:
Step 1: 在Vue组件中引入图片和文本
在Vue组件的template中,使用<img>
标签引入图片,并使用<p>
标签或其他适当的标签来添加文本。
<template>
<div>
<img src="your-image-url" alt="your-image-description" />
<p>your-text</p>
</div>
</template>
Step 2: 样式设置
为了使图片和文字在同一行显示,可以使用CSS的display: flex
属性,并通过justify-content
和align-items
属性来设置图片和文本的对齐方式。
<style>
div {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
</style>
Step 3: 添加动态数据
如果你想在Vue中动态地显示不同的图片和文字,可以使用Vue的数据绑定功能。在Vue组件的data
属性中定义一个对象,包含图片和文本的相关信息。然后在template中使用双括号语法(Mustache语法)来绑定数据。
<template>
<div>
<img :src="image" :alt="altText" />
<p>{{ text }}</p>
</div>
</template>
<script>
export default {
data() {
return {
image: "your-image-url",
altText: "your-image-description",
text: "your-text",
};
},
};
</script>
通过以上步骤,你就可以在Vue中实现图片下方添加文字的效果了。
2. 如何在Vue中实现图片下方添加多个文字效果?
如果你想要在图片下方添加多个文字效果,可以通过以下步骤进行操作:
Step 1: 使用HTML标签包裹文本
在template中使用适当的HTML标签(如<div>
、<span>
等)来包裹每个文本,使其成为独立的元素。
<template>
<div>
<img src="your-image-url" alt="your-image-description" />
<div>
<span>your-text1</span>
<span>your-text2</span>
<span>your-text3</span>
</div>
</div>
</template>
Step 2: 样式设置
为了使每个文本元素在同一行显示,可以使用CSS的display: flex
属性,并通过justify-content
和align-items
属性来设置文本的对齐方式。
<style>
div {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
div > div {
display: flex;
justify-content: center;
align-items: center;
}
div > div > span {
margin: 0 10px; /* 可根据实际需求调整间距 */
}
</style>
Step 3: 添加动态数据
如果你想在Vue中动态地显示不同的文本,可以使用Vue的数据绑定功能。在Vue组件的data
属性中定义一个数组,包含每个文本的相关信息。然后在template中使用v-for
指令来遍历数组并显示每个文本。
<template>
<div>
<img src="your-image-url" alt="your-image-description" />
<div>
<span v-for="text in texts">{{ text }}</span>
</div>
</div>
</template>
<script>
export default {
data() {
return {
texts: ["your-text1", "your-text2", "your-text3"],
};
},
};
</script>
通过以上步骤,你就可以在Vue中实现图片下方添加多个文字的效果了。
3. 如何在Vue中实现图片下方添加文字的动画效果?
如果你希望在Vue中实现图片下方添加文字的动画效果,可以通过以下步骤进行操作:
Step 1: 使用Vue的过渡效果
Vue提供了过渡效果的内置组件<transition>
,可以用来包裹要添加动画效果的元素。在template中使用<transition>
标签包裹图片和文字元素。
<template>
<div>
<transition name="fade">
<img src="your-image-url" alt="your-image-description" />
<p>{{ text }}</p>
</transition>
</div>
</template>
Step 2: 定义过渡效果的CSS样式
在style标签中定义过渡效果的CSS样式。可以使用Vue的过渡类名,如.fade-enter
、.fade-leave-to
等,来指定动画效果的起始和结束状态。
<style>
.fade-enter-active,
.fade-leave-active {
transition: opacity 0.5s;
}
.fade-enter,
.fade-leave-to {
opacity: 0;
}
</style>
Step 3: 添加动态数据和触发动画效果
在Vue组件的data
属性中定义一个变量,用来控制是否显示图片和文字。通过改变这个变量的值,来触发过渡效果。
<template>
<div>
<transition name="fade">
<img v-if="showImage" src="your-image-url" alt="your-image-description" />
<p v-if="showText">{{ text }}</p>
</transition>
<button @click="toggleAnimation">Toggle Animation</button>
</div>
</template>
<script>
export default {
data() {
return {
showImage: false,
showText: false,
text: "your-text",
};
},
methods: {
toggleAnimation() {
this.showImage = !this.showImage;
this.showText = !this.showText;
},
},
};
</script>
通过以上步骤,你就可以在Vue中实现图片下方添加文字的动画效果了。点击按钮时,图片和文字将会显示或隐藏,并带有过渡动画效果。
文章标题:vue如何图片下加文字,发布者:worktile,转载请注明出处:https://worktile.com/kb/p/3603989
赞 (0)
打赏
微信扫一扫
支付宝扫一扫
如果需要更复杂的布局和样式控制,可以使用<div>
和<span>
标签,通过CSS进行样式调整。
<template>
<div class="image-container">
<img src="path/to/your/image.jpg" alt="描述图片的文字">
<span class="image-caption">这是图片下方的文字说明</span>
</div>
</template>
<style scoped>
.image-container {
text-align: center;
margin: 20px;
position: relative;
}
.image-caption {
display: block;
margin-top: 10px;
font-size: 16px;
color: #555;
}
</style>
三、通过CSS定位和样式控制
在需要更复杂的布局时,可以使用CSS定位和样式控制,例如绝对定位或Flexbox布局。
<template>
<div class="image-wrapper">
<img src="path/to/your/image.jpg" alt="描述图片的文字">
<div class="caption">这是图片下方的文字说明</div>
</div>
</template>
<style scoped>
.image-wrapper {
position: relative;
text-align: center;
margin: 20px;
}
img {
width: 100%;
height: auto;
}
.caption {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
background-color: rgba(0, 0, 0, 0.5);
color: #fff;
padding: 5px 10px;
border-radius: 5px;
}
</style>
四、使用Vue组件化处理
为了更好的复用和维护,可以将图文显示的部分封装成一个Vue组件。
<template>
<div class="image-text">
<img :src="imageSrc" :alt="imageAlt">
<p>{{ caption }}</p>
</div>
</template>
<script>
export default {
props: {
imageSrc: {
type: String,
required: true
},
imageAlt: {
type: String,
default: ''
},
caption: {
type: String,
required: true
}
}
}
</script>
<style scoped>
.image-text {
text-align: center;
margin: 20px;
}
img {
width: 100%;
height: auto;
}
p {
margin-top: 10px;
font-size: 16px;
color: #555;
}
</style>
使用这个组件时,可以在父组件中传递图片和文字的参数:
<template>
<div>
<ImageText
imageSrc="path/to/your/image.jpg"
imageAlt="描述图片的文字"
caption="这是图片下方的文字说明"
/>
</div>
</template>
<script>
import ImageText from './components/ImageText.vue';
export default {
components: {
ImageText
}
}
</script>
总结:在Vue中实现图片下方添加文字,可以通过使用<figure>
和<figcaption>
标签、利用<div>
和<span>
标签、以及通过CSS定位和样式控制等方式来实现。选择合适的方法可以根据具体需求和项目的复杂度来决定。为了更好的代码复用性和可维护性,可以将图文显示部分封装成Vue组件。
相关问答FAQs:
1. 如何在Vue中实现图片下方添加文字效果?
在Vue中,要实现在图片下方添加文字的效果,可以通过以下步骤进行操作:
Step 1: 在Vue组件中引入图片和文本
在Vue组件的template中,使用<img>
标签引入图片,并使用<p>
标签或其他适当的标签来添加文本。
<template>
<div>
<img src="your-image-url" alt="your-image-description" />
<p>your-text</p>
</div>
</template>
Step 2: 样式设置
为了使图片和文字在同一行显示,可以使用CSS的display: flex
属性,并通过justify-content
和align-items
属性来设置图片和文本的对齐方式。
<style>
div {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
</style>
Step 3: 添加动态数据
如果你想在Vue中动态地显示不同的图片和文字,可以使用Vue的数据绑定功能。在Vue组件的data
属性中定义一个对象,包含图片和文本的相关信息。然后在template中使用双括号语法(Mustache语法)来绑定数据。
<template>
<div>
<img :src="image" :alt="altText" />
<p>{{ text }}</p>
</div>
</template>
<script>
export default {
data() {
return {
image: "your-image-url",
altText: "your-image-description",
text: "your-text",
};
},
};
</script>
通过以上步骤,你就可以在Vue中实现图片下方添加文字的效果了。
2. 如何在Vue中实现图片下方添加多个文字效果?
如果你想要在图片下方添加多个文字效果,可以通过以下步骤进行操作:
Step 1: 使用HTML标签包裹文本
在template中使用适当的HTML标签(如<div>
、<span>
等)来包裹每个文本,使其成为独立的元素。
<template>
<div>
<img src="your-image-url" alt="your-image-description" />
<div>
<span>your-text1</span>
<span>your-text2</span>
<span>your-text3</span>
</div>
</div>
</template>
Step 2: 样式设置
为了使每个文本元素在同一行显示,可以使用CSS的display: flex
属性,并通过justify-content
和align-items
属性来设置文本的对齐方式。
<style>
div {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
div > div {
display: flex;
justify-content: center;
align-items: center;
}
div > div > span {
margin: 0 10px; /* 可根据实际需求调整间距 */
}
</style>
Step 3: 添加动态数据
如果你想在Vue中动态地显示不同的文本,可以使用Vue的数据绑定功能。在Vue组件的data
属性中定义一个数组,包含每个文本的相关信息。然后在template中使用v-for
指令来遍历数组并显示每个文本。
<template>
<div>
<img src="your-image-url" alt="your-image-description" />
<div>
<span v-for="text in texts">{{ text }}</span>
</div>
</div>
</template>
<script>
export default {
data() {
return {
texts: ["your-text1", "your-text2", "your-text3"],
};
},
};
</script>
通过以上步骤,你就可以在Vue中实现图片下方添加多个文字的效果了。
3. 如何在Vue中实现图片下方添加文字的动画效果?
如果你希望在Vue中实现图片下方添加文字的动画效果,可以通过以下步骤进行操作:
Step 1: 使用Vue的过渡效果
Vue提供了过渡效果的内置组件<transition>
,可以用来包裹要添加动画效果的元素。在template中使用<transition>
标签包裹图片和文字元素。
<template>
<div>
<transition name="fade">
<img src="your-image-url" alt="your-image-description" />
<p>{{ text }}</p>
</transition>
</div>
</template>
Step 2: 定义过渡效果的CSS样式
在style标签中定义过渡效果的CSS样式。可以使用Vue的过渡类名,如.fade-enter
、.fade-leave-to
等,来指定动画效果的起始和结束状态。
<style>
.fade-enter-active,
.fade-leave-active {
transition: opacity 0.5s;
}
.fade-enter,
.fade-leave-to {
opacity: 0;
}
</style>
Step 3: 添加动态数据和触发动画效果
在Vue组件的data
属性中定义一个变量,用来控制是否显示图片和文字。通过改变这个变量的值,来触发过渡效果。
<template>
<div>
<transition name="fade">
<img v-if="showImage" src="your-image-url" alt="your-image-description" />
<p v-if="showText">{{ text }}</p>
</transition>
<button @click="toggleAnimation">Toggle Animation</button>
</div>
</template>
<script>
export default {
data() {
return {
showImage: false,
showText: false,
text: "your-text",
};
},
methods: {
toggleAnimation() {
this.showImage = !this.showImage;
this.showText = !this.showText;
},
},
};
</script>
通过以上步骤,你就可以在Vue中实现图片下方添加文字的动画效果了。点击按钮时,图片和文字将会显示或隐藏,并带有过渡动画效果。
文章标题:vue如何图片下加文字,发布者:worktile,转载请注明出处:https://worktile.com/kb/p/3603989
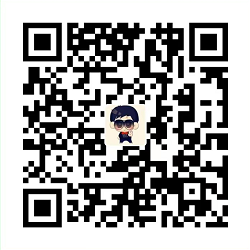
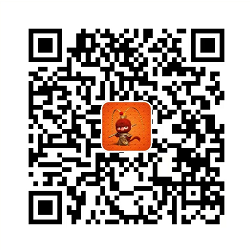